Lab 02: Currency conversion
This lab will exercise your skill with if
statements in dealing with currency conversions. Some useful references will be the Unit 2 notes and, as always, the C functions reference for this class.
Submit in the normal way, being sure to put your files in a directory called lab02
.
1 Converting Currencies
Write a program conv1.c
that converts between Dollars, Euros and Yen. The program reads input from the user in the following format: Convert <amount> <currency1> to <currency2>
and prints out the resulting currency amount. Here are a couple of sample runs:
roche@ubuntu$
./conv1
Convert 3.50 Euros to Dollars
3.76344
roche@ubuntu$
./conv1
Convert 350 Yen to Euros
2.83982
Here are the conversion rates you’ll need: 1.00 Dollar is 0.93 Euros and 1.00 Dollar is 114.62 Yen.
2 Two-word currencies
You are going to extend your program from Part 1 into a new file called conv2.c
. Important: don’t delete your working Part 1 conv1.c
file! Once you have part 1 working perfectly, just copy that file using a command like
roche@ubuntu$
cp conv1.c conv2.c
and then get to work on this part under the new filename.
Your conv2.c
program will allow for Canadian dollars as well as the other currencies. Now the user can’t simply put “Dollars” in the input, it must be either “Dollars US” or “Dollars Canadian”. Here are a couple of sample runs:
roche@ubuntu$
./conv2
Convert 3.50 Euros to Dollars Canadian
5.00538
roche@ubuntu$
./conv2
Convert 11.72 Dollars US to Dollars Canadian
15.5876
roche@ubuntu$
./conv2
Convert 350 Yen to Euros
2.83982
You’ll be interested to know that 1.00 Dollars US is 1.33 Dollars Canadian.
3 Adding multiple currencies (going further)
The rest of this lab is optional for your enrichment and enjoyment. Be sure to work on this only after getting everything else perfect. You should still submit this work, but make sure it doesn't break any of the parts you already had working correctly!
Copy your program to a new file called conv3.c
for this part.
Extend your program again so that, in addition to dealing with Dollars US
and Dollars Canadian
, the user may also add up two currencies to convert by using the word and
. However, note that they should still be able to do just one currency like before. For example:
roche@ubuntu$
./conv3
Convert 3.50 Euros and 2 Dollars US to Dollars Canadian
7.66538
roche@ubuntu$
./conv3
Convert 11.72 Dollars US to Dollars Canadian
15.5876
roche@ubuntu$
./conv3
Convert 1 Dollars Canadian and 8 Dollars Canadian to Yen
775.624
4 Classifying triangles (going even further)
There are two common ways of classifying a triangle:
- By describing its interior angles
- Acute triangles have three interior angles that are all less than 90 degrees
- Right triangles have an interior angle that is exactly 90 degrees
- Obtuse triangles have an interior angle that is greater than 90 degrees
- By describing the lengths of the sides in relation to each other
- Equilateral triangles have three sides that are all of equal length
- Isosceles triangles have two sides that are of equal length
- Scalene triangle have all three sides of different lengths
A well known property of all triangles is that the sum of any two sides will always be greater than the third, a fact known as the triangle inequality. Given a set of three lengths it is possible to determine if they can form a triangle by adding two lengths together and comparing them to the third.
Given the three lengths of the sides of a triangle, a, b and c, it is also possible to calculate the area of a triangle using Heron’s formula.
\[ \Delta = \sqrt{s(s-a)(s-b)(s-c)} \mbox{, where } s = \frac{1}{2}(a + b + c) \]
Also, from the side lengths \(a\), \(b\) and \(c\), it is possible to calculate the three interior angles, \(A\), \(B\) and \(C\), of the triangle using the law of sines:
\[ \frac{sin{A}}{a} = \frac{sin{B}}{b} = \frac{sin{C}}{c} = \frac{2\Delta}{abc}. \]
Your job: write a program triangles.c
that reads three lengths from the user displays the type of triangle that is formed by the input according to both kinds of classification. E.g. “This is an obtuse scalene triangle”
roche@ubuntu$
./triangles
Enter side lengths:
1 2 3
These lengths violate the triangle inequality!
roche@ubuntu$
./triangles
Enter side lengths:
1.5 2 3
This is an obtuse scalene triangle.
roche@ubuntu$
./triangles
Enter side lengths:
2 2 3
This is an obtuse isosceles triangle.
roche@ubuntu$
./triangles
Enter side lengths:
3 4 5
This is a right scalene triangle.
roche@ubuntu$
./triangles
Enter side lengths:
2 2 2
This is an acute equilateral triangle.
roche@ubuntu$
./triangles
Enter side lengths:
2 2 1.5
This is an acute isosceles triangle.
Tips: The math.h
library has a function asin()
that computes the arcsine of an angle. Enter man asin
on the command line for documentation. We have a bit of a problem in that for any positive angle \(\theta\) less than \(\pi/2\), we have that \(\sin(\theta) = \sin(\pi - \theta)\). Thus, when we use \(\theta\) = asin(w)
for positive w
, we don’t know whether we really want the angle \(\theta\) or \(\pi - \theta\). In our triangle case, we can fix things like this: if the three angles we calculate (A, B and C) sum up to a lot less than \(\pi\) (in fact, we should probably check that the sum is less than 3), we replace the angle opposite the longest side with \(\pi\) minus that angle. So, for example, if \(A\) is opposite the longest side, then we replace \(A\) with \(\pi - A\).
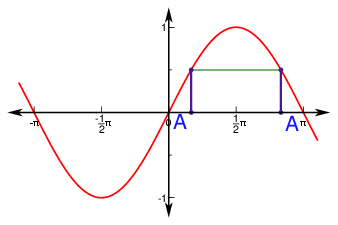
BTW: as long as you’re including math.h
, the constant M_PI
gives you a high-precision double representation of \(\pi\).
Also: the function sqrt( )
computes square roots.
Also: Don’t forget to add -lm
to the end of your gcc
command in order to compile with the math library support.